参考
思想:把对象创建和调用交给spring管理,降低耦合
实现:配置解析->工厂->创建对象(反射)->DI(注入属性)
beans(演员)
创建
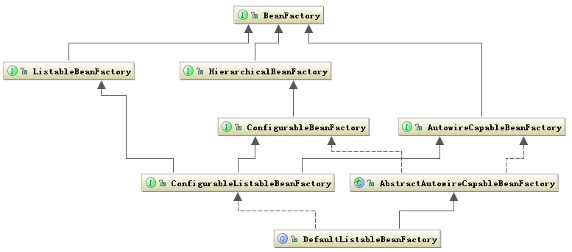
- BeanFactory:IOC容器基本实现,懒加载
- ListableBeanFactory:Bean是可列表的
- HierarchicalBeanFactory:Bean有继承关系
- AutowireCapableBeanFactory:Bean的自动装配规则
- DefaultListableBeanFactory:默认实现
定义
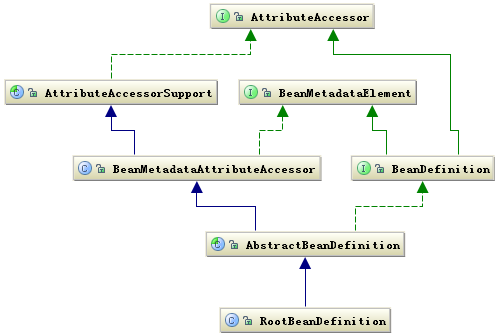
解析
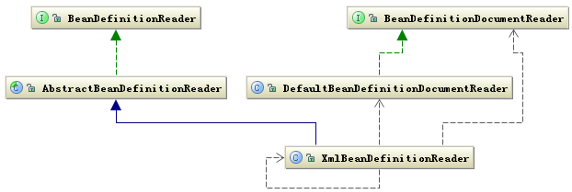
context(舞台)
给Spring提供运行时的环境(Ioc容器),用以保存各个对象的状态。整合了大部分功能
Context类图
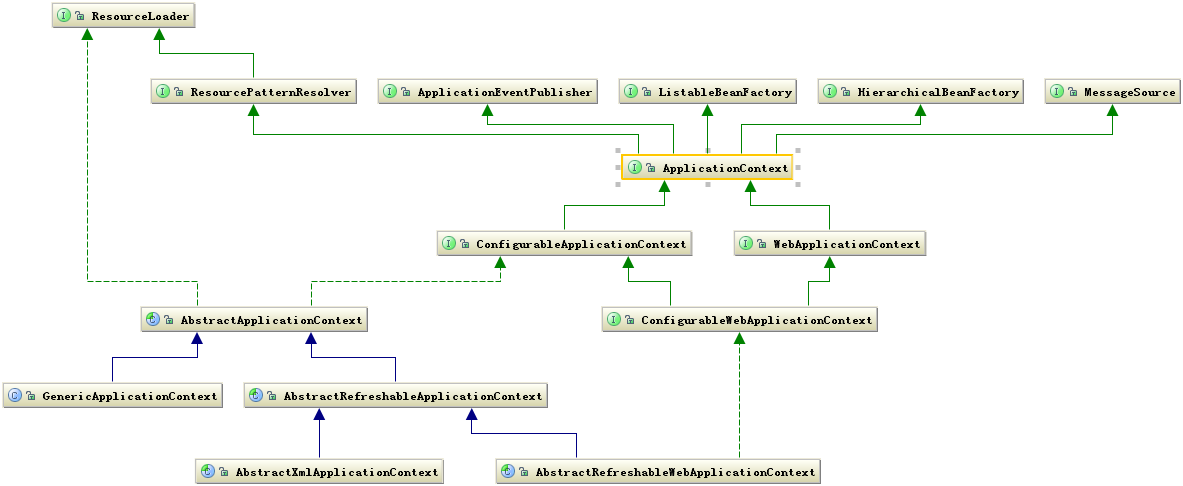
- ResourceLoader:访问外部资源
- ConfigurableApplicationContext:可动态添加或修改已有的配置信息
- AbstractRefreshableApplicationContext(常用)
作用
- 标识应用环境
- 创建Bean对象
- 保存对象关系表
- 捕获各种事件
core(道具)
定义了资源的访问方式
Resource类图
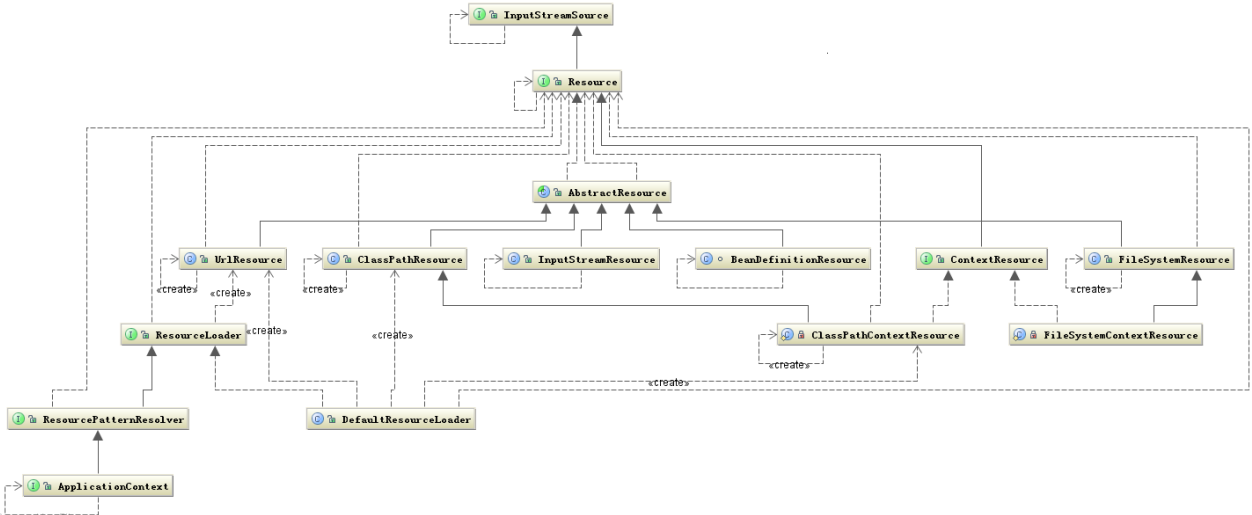
Context和Resource类关系图
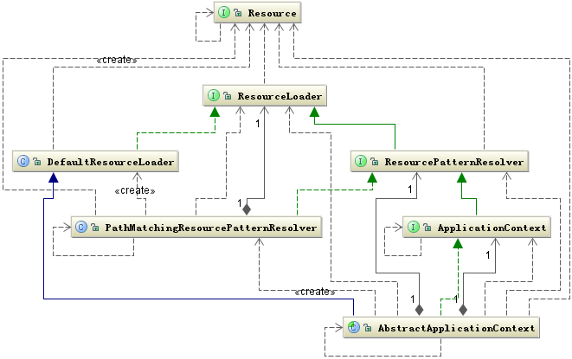
ioc
AbstractApplicationContext
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
| public void refresh() throws BeansException, IllegalStateException {
synchronized (this.startupShutdownMonitor) {
// Prepare this context for refreshing.
prepareRefresh();
// Tell the subclass to refresh the internal bean factory.
ConfigurableListableBeanFactory beanFactory = obtainFreshBeanFactory();//创建BeanFactory
// Prepare the bean factory for use in this context.
prepareBeanFactory(beanFactory);//配置BeanFactory:添加一些Spring本身需要的一些工具类
try {
// Allows post-processing of the bean factory in context subclasses.
postProcessBeanFactory(beanFactory);
// Invoke factory processors registered as beans in the context.
invokeBeanFactoryPostProcessors(beanFactory);//对已经构建的 BeanFactory 的配置做修改(实现BeanFactoryPostProcessor接口)
// Register bean processors that intercept bean creation.
registerBeanPostProcessors(beanFactory);//对以后再创建 Bean 的实例对象时添加一些自定义的操作(实现BeanPostProcessor接口)
// Initialize message source for this context.
initMessageSource();
// Initialize event multicaster for this context.
initApplicationEventMulticaster();//初始化监听事件
// Initialize other special beans in specific context subclasses.
onRefresh();
// Check for listener beans and register them.
registerListeners();//对系统的其他监听者的注册(实现ApplicationListener接口)
// Instantiate all remaining (non-lazy-init) singletons.
finishBeanFactoryInitialization(beanFactory);//创建 Bean 实例并构建 Bean 的关系网
// Last step: publish corresponding event.
finishRefresh();
} catch (BeansException ex) {
// Destroy already created singletons to avoid dangling resources.
destroyBeans();
// Reset 'active' flag.
cancelRefresh(ex);
// Propagate exception to caller.
throw ex;
} finally {
// Reset common introspection caches in Spring's core, since we
// might not ever need metadata for singleton beans anymore...
resetCommonCaches();
}
}
}
|
构建BeanFactory
AbstractRefreshableApplicationContext
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
| protected final void refreshBeanFactory() throws BeansException {
if (hasBeanFactory()) {
destroyBeans();
closeBeanFactory();
}
try {
DefaultListableBeanFactory beanFactory = createBeanFactory();//BeanFactory的原始对象
beanFactory.setSerializationId(getId());
customizeBeanFactory(beanFactory);
loadBeanDefinitions(beanFactory);//加载、解析Bean的定义
this.beanFactory = beanFactory;
} catch (IOException ex) {
throw new ApplicationContextException("I/O error parsing bean definition source for " + getDisplayName(), ex);
}
}
|
DefaultListableBeanFactory
类图
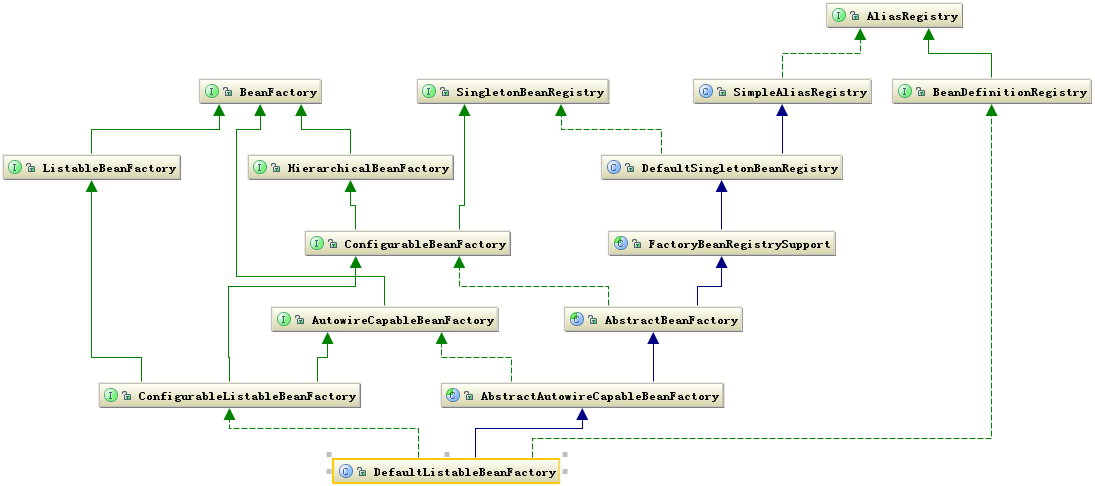
Bean的实例化
DefaultListableBeanFactory
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
| public void preInstantiateSingletons() throws BeansException {
// Iterate over a copy to allow for init methods which in turn register new bean definitions.
// While this may not be part of the regular factory bootstrap, it does otherwise work fine.
List<String> beanNames = new ArrayList<>(this.beanDefinitionNames);
// Trigger initialization of all non-lazy singleton beans...
for (String beanName : beanNames) {
RootBeanDefinition bd = getMergedLocalBeanDefinition(beanName);
if (!bd.isAbstract() && bd.isSingleton() && !bd.isLazyInit()) {
if (isFactoryBean(beanName)) {
Object bean = getBean(FACTORY_BEAN_PREFIX + beanName);
if (bean instanceof FactoryBean) {
FactoryBean<?> factory = (FactoryBean<?>) bean;
boolean isEagerInit;
if (System.getSecurityManager() != null && factory instanceof SmartFactoryBean) {
isEagerInit = AccessController.doPrivileged(
(PrivilegedAction<Boolean>) ((SmartFactoryBean<?>) factory)::isEagerInit,
getAccessControlContext());
} else {
isEagerInit = (factory instanceof SmartFactoryBean &&
((SmartFactoryBean<?>) factory).isEagerInit());
}
if (isEagerInit) {
getBean(beanName);
}
}
} else {
getBean(beanName);//创建实例
}
}
}
}
|
AbstractAutowireCapableBeanFactory
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| protected Object doCreateBean(String beanName, RootBeanDefinition mbd, @Nullable Object[] args) throws BeanCreationException {
// Instantiate the bean.
BeanWrapper instanceWrapper = null;
if (instanceWrapper == null) {
instanceWrapper = createBeanInstance(beanName, mbd, args);//实例化阶段
}
// Initialize the bean instance.
Object exposedObject = bean;
try {
populateBean(beanName, mbd, instanceWrapper);//属性赋值阶段
exposedObject = initializeBean(beanName, exposedObject, mbd);//初始化阶段
}
}
|
扩展点
第一大类:影响多个Bean的接口
InstantiationAwareBeanPostProcessor
作用于实例化阶段的前后
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
| @Override
protected Object createBean(String beanName, RootBeanDefinition mbd, @Nullable Object[] args) throws BeanCreationException {
try {
// Give BeanPostProcessors a chance to return a proxy instead of the target bean instance.
Object bean = resolveBeforeInstantiation(beanName, mbdToUse);// postProcessBeforeInstantiation方法调用点
if (bean != null) {
return bean;
}
}
try {
Object beanInstance = doCreateBean(beanName, mbdToUse, args);
if (logger.isTraceEnabled()) {
logger.trace("Finished creating instance of bean '" + beanName + "'");
}
return beanInstance;
}
}
protected void populateBean(String beanName, RootBeanDefinition mbd, @Nullable BeanWrapper bw) {
// Give any InstantiationAwareBeanPostProcessors the opportunity to modify the
// state of the bean before properties are set. This can be used, for example,
// to support styles of field injection.
boolean continueWithPropertyPopulation = true;
if (!mbd.isSynthetic() && hasInstantiationAwareBeanPostProcessors()) {
for (BeanPostProcessor bp : getBeanPostProcessors()) {
if (bp instanceof InstantiationAwareBeanPostProcessor) {
InstantiationAwareBeanPostProcessor ibp = (InstantiationAwareBeanPostProcessor) bp;
if (!ibp.postProcessAfterInstantiation(bw.getWrappedInstance(), beanName)) {// postProcessAfterInstantiation方法调用点
continueWithPropertyPopulation = false;
break;
}
}
}
}
}
|
BeanPostProcessor
作用于初始化阶段的前后
执行顺序:PriorityOrdered > Ordered
第二大类:只调用一次的接口
Aware
让我们能够拿到Spring容器中的一些资源
Aware Group1
- BeanNameAware
- BeanClassLoaderAware
- BeanFactoryAware
Aware Group2
- EnvironmentAware
- EmbeddedValueResolverAware:实现该接口能够获取Spring EL解析器,用户的自定义注解需要支持spel表达式的时候使用
- ApplicationContextAware(ResourceLoaderAware\ApplicationEventPublisherAware\MessageSourceAware)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
| // 初始化阶段
protected Object initializeBean(final String beanName, final Object bean, @Nullable RootBeanDefinition mbd) {
invokeAwareMethods(beanName, bean);// Group1中的三个Bean开头的Aware
Object wrappedBean = bean;
// 这里调用的是Group2中的几个Aware,
// 而实质上这里就是前面所说的BeanPostProcessor的调用点!
// 这里是通过BeanPostProcessor(ApplicationContextAwareProcessor)实现的。
wrappedBean = applyBeanPostProcessorsBeforeInitialization(wrappedBean, beanName);
invokeInitMethods(beanName, wrappedBean, mbd);// InitializingBean调用点
wrappedBean = applyBeanPostProcessorsAfterInitialization(wrappedBean, beanName);// BeanPostProcessor的另一个调用点
return wrappedBean;
}
|
生命周期接口
InitializingBean
:对应生命周期的初始化阶段
DisposableBean
:对应生命周期的销毁阶段,以ConfigurableApplicationContext#close()方法作为入口
文章作者
lim
上次更新
2024-11-21
(1dac9ff)
许可协议
CC BY-NC-ND 4.0